1일 1개념정리 24.08.09.금 ~
큰 결정에 큰 동기가 따르지 않을 때도 있다. 하지만 큰 결심이 따라야 이뤄낼 수 있다.
무조건 무조건 1일 1개의 개념 정리하기 !!!!!!!!!!!!!!!!!!!!!!!!!!!!!
#19. static
static은 자바에서 클래스 레벨의 변수나 메소드를 다룰 때 사용한다. 이는 클래스의 인스턴스가 아닌, 클래스 자체에 속하는 멤버임을 뜻한다. static은 클래스와 관련있다고 생각하면 편하다.
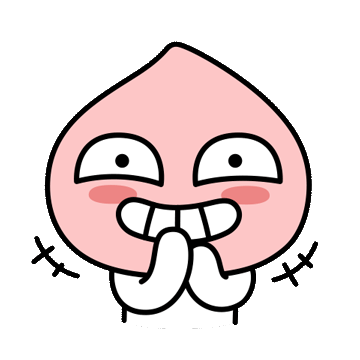
Static 변수 (클래스 변수)
- 정의 : static 변수는 클래스의 모든 인스턴스가 공유하는 변수이다. 이는 클래스가 메모리에 로드될 때 초기화되고 프로그램이 종료될 때까지 유지된다.
- 사용 : 일반적으로 공통된 값을 여러 객체가 공유해야 할 때 사용한다.
class Example {
static int count = 0; // 클래스 변수
// 객체 생성할 때 count 증가
Example() {
count++;
}
}
public class Main {
public static void main(String[] args) {
Example obj1 = new Example();
Example obj2 = new Example();
System.out.println(Example.count); // 출력 : 2
}
}
Static 메소드 (클래스 메소드)
- 정의 : static 메소드는 객체 인스턴스와 무관하게 호출할 수 있다. 즉, 객체를 생성하지 않고도 클래스 이름을 통해 직접 호출이 가능하다.
- 제한 : static 메소드는 인스턴스 변수나 인스턴스 메소드를 직접 사용할 수 없다. 이는 static 메소드가 인스턴스와 무관하게 동작하기 때문입니다. (아래 Example2 예시)
class Example {
static int count = 0;
static void incrementCount() { // 클래스 메소드
count++;
}
}
public class Main {
public static void main(String[] args) {
Example.incrementCount(); // 객체 생성 없이도 클래스명으로 직접 호출 가능
System.out.println(Example.count); // 출력: 1
}
}
//////////////////////////////////
// 제한사항 예시
class Example2 {
int instanceVar = 42; // 인스턴스 변수
void instanceMethod() { // 인스턴스 메소드
System.out.println("This is an instance method.");
}
static void staticMethod() {
// 인스턴스 변수 접근 - 오류
// System.out.println(instanceVar); // 오류 : 인스턴스 변수 참조 불가
// 인스턴스 메소드 호출 - 오류
// instanceMethod(); // 오류 : 인스턴스 메소드 참조 불가
System.out.println("This is a static method.");
}
}
Static 클래스
- 정의 : static 클래스는 static으로 선언된 중첩 클래스(내부 클래스)를 의미한다. 이 클래스는 외부 클래스의 인스턴스에 속하지 않고 독립적으로 동작할 수 있다. 일반적으로 static 중첩 클래스는 외부 클래스와 관련이 있지만, 외부 클래스의 인스턴스에 접근할 필요가 없는 경우에 사용된다.
class OuterClass {
static int outerStaticVar = 10;
int outerInstanceVar = 20;
// static 중첩 클래스
static class StaticNestedClass {
void display() {
// 외부 클래스의 static 변수에 접근 가능
System.out.println("Outer static variable: " + outerStaticVar);
// 외부 클래스의 인스턴스 변수에 접근 불가
// System.out.println("Outer instance variable: " + outerInstanceVar); // 오류 발생
System.out.println("This is a static nested class.");
}
}
// 일반 중첩 클래스 (static이 아닌 경우)
class NonStaticNestedClass {
void display() {
// 외부 클래스의 static 변수 및 인스턴스 변수에 모두 접근 가능
System.out.println("Outer static variable: " + outerStaticVar);
System.out.println("Outer instance variable: " + outerInstanceVar);
System.out.println("This is a non-static nested class.");
}
}
}
public class Main {
public static void main(String[] args) {
// Static 중첩 클래스의 객체 생성
OuterClass.StaticNestedClass staticNestedObj = new OuterClass.StaticNestedClass();
staticNestedObj.display(); // 호출 : Outer static variable: 10 / This is a static nested class.
// Non-static 중첩 클래스의 객체 생성 (외부 클래스의 인스턴스가 필요함)
OuterClass outerObj = new OuterClass();
OuterClass.NonStaticNestedClass nonStaticNestedObj = outerObj.new NonStaticNestedClass();
nonStaticNestedObj.display(); // 호출 : Outer static variable: 10 / Outer instance variable: 20 / This is a non-static nested class.
}
}
Static 블록
- 정의 : static 블록은 클래스가 메모리에 로드될 때만 딱 한 번 실행되는 코드 블록이다. static 변수를 초기화할 대 사용할 수 있다.
class Example {
static int count;
// static 블록
static {
count = 10;
System.out.println("Static block called.");
}
}
public class Main {
public static void main(String[] args) {
System.out.println(Example.count); // 출력 : 10
}
}
시험 끝나고 보충하기.
'1일 1개념정리 (24년 8월~) > Java' 카테고리의 다른 글
1일1개 (21) - 불변객체란 ? (0) | 2024.08.30 |
---|---|
1일1개 (20) - ==와 equals()의 차이 (0) | 2024.08.29 |
1일1개 (18) - 상속과 구현 (0) | 2024.08.27 |
1일1개 (17) - 오버라이딩 vs 오버로딩 (0) | 2024.08.26 |
1일1개 (16) - 자바 final (0) | 2024.08.25 |